Introduction to React-three-fiber Part 2
Hello 👋, Welcome to Part 2 of our blog series on react-three-fiber
. In this tutorial, we will learn how to render any 3D model in your scene using gltfjsx
.
If you followed along with Part 1, you already know how to create a basic 3D scene using react-three-fiber
. Now, let's take it to the next level with 3D models!
In case you’re wondering! Check out what we'll be building today at
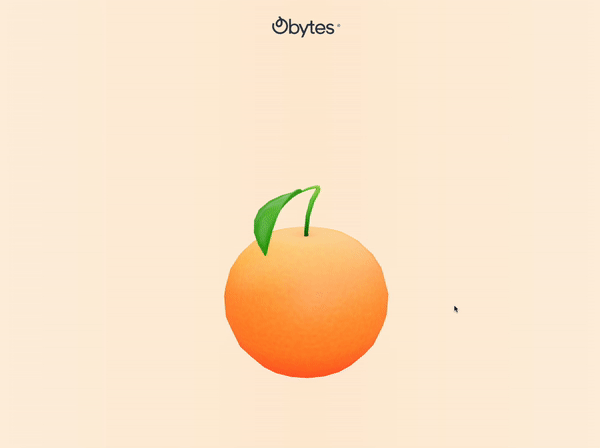
To follow along with this tutorial, you will need a 3D model. We will be using the orange 3D model built by bariacg, which you can download glTF
format for free and copy textures, scene.bin
 and scene.gltf into your public folder.
Firstly, let's understand what is gltfjsx
gltfjsx
is a tool that generates React
components from glTF
files format for 3D scenes and models using the JSON standard.
Before starting, we need to install react-three/fiber
and react-three/drei
using the following command:
npm i @react-three/fiber
npm install @react-three/drei
Once installed, we can convert our glTF
file to a React
component using the following command using gltfjsx
:
npx gltfjsx scene.gltf
This will generate a React
component for our 3D model. We can then import this component and use it in our scene.
import { useGLTF } from '@react-three/drei';
import React from 'react';
export function Model(props) {
const { nodes, materials } = useGLTF('/scene.gltf');
return (
<group {...props} dispose={null}>
<mesh
geometry={nodes.Orange_Orange_0.geometry}
material={materials.Orange}
scale={1.5}
/>
</group>
);
}
useGLTF.preload('/scene.gltf');
Now that we have our Model
component ready, we can import it within a react-three-fiber
canvas to handle the scene creation!
In order to make our 3D model impressive and ready for display on the web, we will add the ambientLight
and OrbitControls
components. With these additions, our 3D model will be fully interactive and ready to be explored by users.
import { Model } from './Components/Model';
import { OrbitControls } from '@react-three/drei';
import { Canvas } from '@react-three/fiber';
function App() {
return (
<Canvas
camera={{
position: [0, 50, 100],
}}
>
<ambientLight intensity={1.2} />
<Model />
<OrbitControls />
</Canvas>
);
}
export default App;
In conclusion, using gltfjsx
to generate React components from glTF
files makes it easy to render 3D models. By following the steps outlined in this blog, you can render any 3D model in your react-three-fiber
scene. So, if you found this blog helpful and informative, don't hesitate to share it on your social media channels.